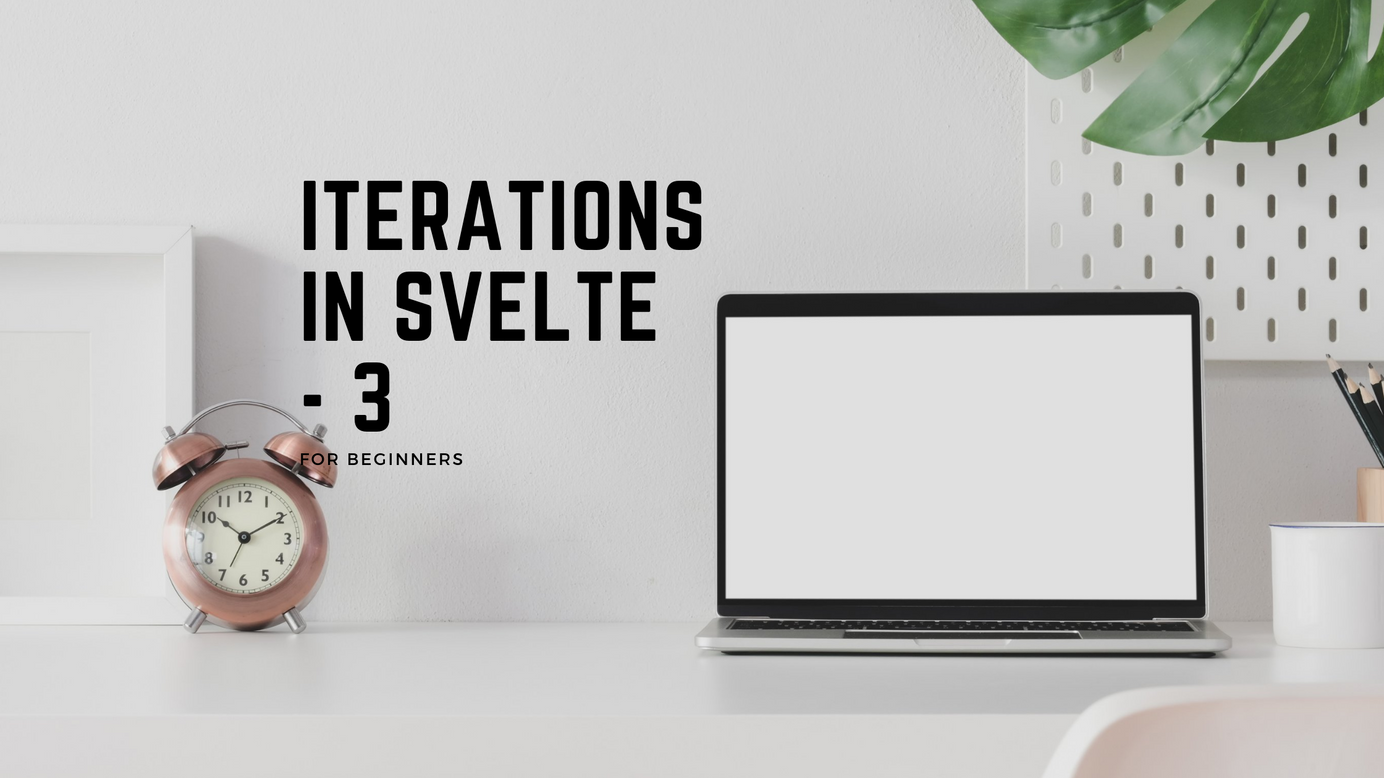
How For Loop works in Svelte?
When we are developing web applications, we need to iterate over items often. Iterations in Svelte are no exception.
Like {#if} iterations in HTML begins with {#each} and ends with {/each}.
The expression that follows {#each} can be any JavaScript expression.
Optionally {:else} can be used before {/each}. Content after {:else} is rendered when the array is empty.
Let's say we have an array of fruits, ['Apple', 'Banana', 'Oranges']
. The following example outputs each fruit on a separate line.
<script>
let fruits = ['Apple', 'Banana', 'Oranges']
</script>
{#each fruits as fruit}
<div style="color: red">{fruit} </div> <br/>
{/each}
<style>
p {
text-align: center;
padding: 1em;
max-width: 240px;
margin: 0 auto;
}
</style>
On the webpage it will look like this.

Object Iteration
Object iteration is another way of iterating a JavaScript objects (key and value pair). And it is very common to iterate an object in the applications.
// Object under the <script> tag
let person = {
full_name: 'Michael Foo',
country: 'UK',
age: 50,
occupation: 'Work somewhere'
};
// Under the HTML tag
<h1>...Iterating an object...</h1>
<hr/>
{#each Object.entries(person) as [key, value]}
<div>
<span style="color: blue">{key} </span> : {JSON.stringify(value)}
</div>
{/each}
On the webpage it will look like the below screenshot
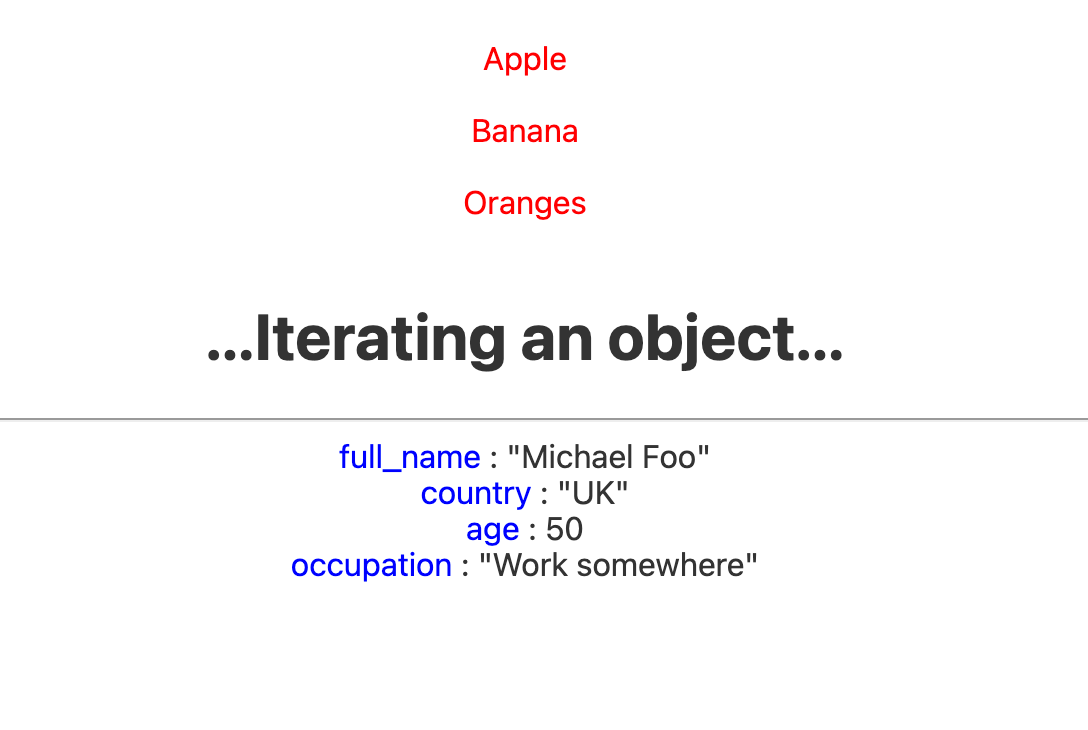
To download the code, please visit
If you want to learn Svelte in detail, you can buy Svelte and Sapper in Action.
PS:- If you purchase from this link, I may get some commission on sales.
AK Newsletter
Join the newsletter to receive the latest updates in your inbox.