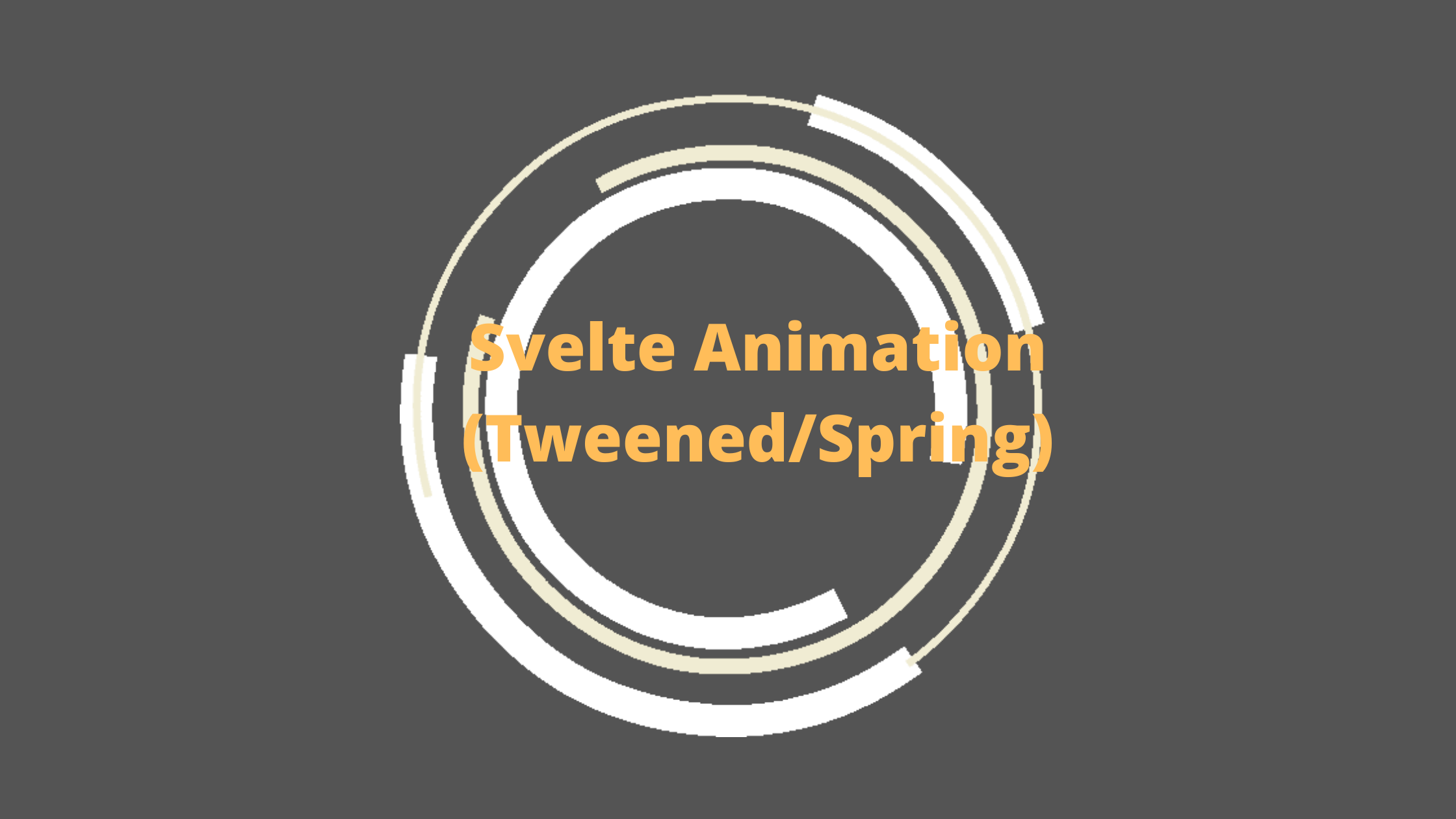
Animation in Svelte/motion ( tweened and spring )
In our previous article, we discuss Animation in Svelte
. If you haven't gone through the article, please visit this link.
We'll start with adding a new route animations/motion
. If you are new here, before proceeding further you need to visit this link. To create a route, we need to create a new file animations/motion.svelte
.
In svelte, there is a library easing library under the svelte. We need to import it like import { tweened, spring } from "svelte/motion"
. And following functions are supported
tweened, spring
We'll create a form and add the three input fields, first_name
, last_name
, and email
. Using the tweened
motion, we'll increase the size of the input fields.
In the new file motion.svelte
start adding the following content:
<script>
import { tweened } from "svelte/motion"
</script>
<hr />
<main style="margin-left: 50%">
<form action="#" >
<label>
First Name <br />
<input type="text" name="first_name">
</label>
<label>
Last Name <br />
<input type="text" name="last_name" >
</label>
<label>
User Email <br />
<input type="email" name="email">
</label>
<input type="submit" name="Submit" >
</form>
</main>
Under script
we only import the package but we haven't used it yet. So lets use the package.
<script>
import { tweened } from "svelte/motion"
import { sineOut } from "svelte/easing"
const size = tweened(25, {
duration: 800,
easing: sineOut
});
</script>
We have imported sineOut
animation function from the svelte/easing
. In the line we use the tweened
function. Remember we only use const
variable for tweened function. Let's write the next line of code
<script>
import { tweened } from "svelte/motion"
import { sineOut } from "svelte/easing"
const size = tweened(25, {
duration: 800,
easing: sineOut
});
function enlargeForm() {
size.set(50)
}
</script>
<hr />
<main style="margin-left: 50%">
<button on:click={enlargeForm}>Enlarge</button>
<br /> <br />
<form action="#" >
<label>
First Name <br />
<input type="text" name="first_name" size="{$size}" >
</label>
<label>
Last Name <br />
<input type="text" name="last_name" size="{$size}" >
</label>
<label>
User Email <br />
<input type="email" name="email" size="{$size}" >
</label>
<input type="submit" name="Submit" >
</form>
</main>
We create the button and bind click event with enlarge
function.
<button on:click={enlargeForm}>Enlarge</button>
And we also defined the enlargeForm
function inside the script
.
function enlargeForm() {
size.set(50)
}
Thats it. We have successfully implemented the tweened
animation. Let's move out to spring
animation.
We'll start with the plain spring
and then add out other affects. Add the following under the script
in motion.svelte
.
const showAndHide = spring(100);
let hide = setInterval(() => {
show.set(0)
}, 500);
let display = setInterval(() => {
show.set(1)
}, 200);
And don't forgot to import spring
import { tweened, spring } from "svelte/motion"
We'll show and hide text Please fill the form
. Add the following under the <main> tag.
{#if $showAndHide >= 1 }
<p style="background-color: #666666; color: white;">
<strong> Please fill this form </strong>
</p>
{/if}
Now go to the browser http://localhost:5000/animations/motion
. You'll see the following.
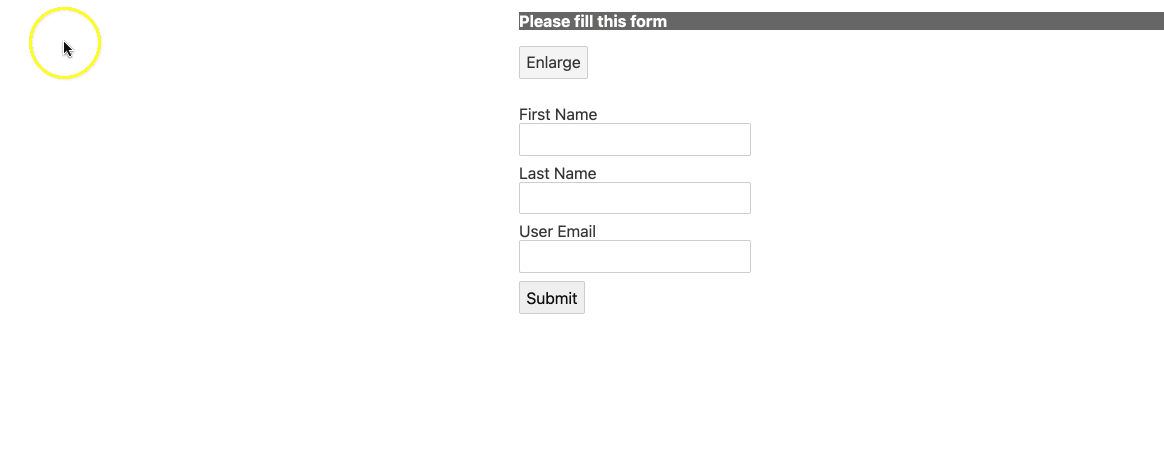
Let's add some stiffness to it. Modify the showAndHide
variable by adding some stiffness :).
const showAndHide = spring(100, {
stiffness: 0.2
});
Save the file and reload the webpage. You'll notice now the blinking is quite nicer than before. Similarly you can play with the damping
effect. Whenever you want to change the image at higher frequency rate, then use spring
and if you want to animate the rows and columns use tweened
.
If you are upto here. Thank you. See you in the next article.
AK Newsletter
Join the newsletter to receive the latest updates in your inbox.