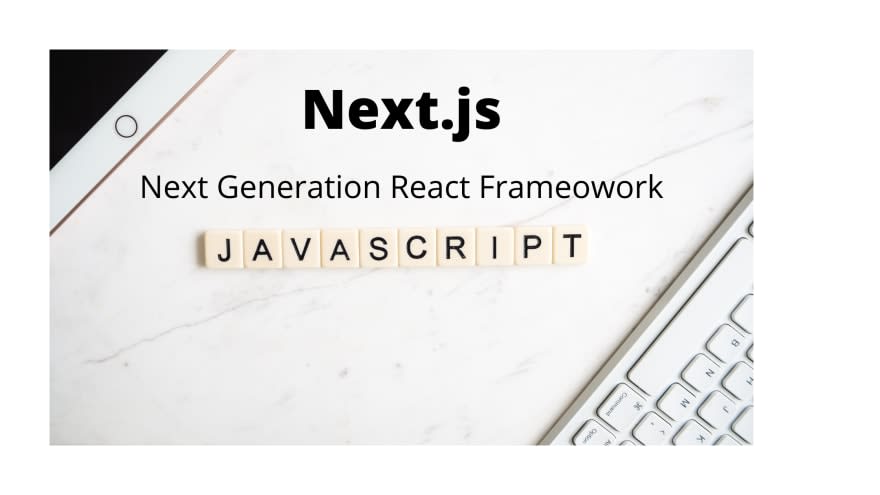
How to Improve SEO in Next.js
In this article, we learn how to make an SEO-friendly Next.js application. First of all, we need to create the Next.js app. If you are new to Next.js, please follow this post to generate Next.js application scaffolding.
In this article, we learn how to make an SEO-friendly Next.js application.
First of all, we need to create the Next.js app. If you are new to Next.js, please follow this post to generate Next.js application scaffolding.
You are here. It's fair to assume you already set up the Next.js skeleton and, your app is running at http://localhost:3000.
At this point, if you do the view-source page, then you see the HTML and CSS. You also see the meta tags, title and, meta description. But if you add a new route to the Next.js app, then you can't see this.
If the route is password protected, it is fair not to add the title and meta-description to the page because it is not visible to the search engine crawlers. But if the SEO matters, then it is a necessity to add title and description.
There are two ways to do it. Open the index.js and search for the following:
<Head>
<title>Create Next App</title>
<meta name="description" content="Generated by create next app" />
<link rel="icon" href="/favicon.ico" />
</Head>
You can copy the code and paste it to every route. And don't forget to add the following code to the top of every route file.
import Head from 'next/head'
There is another way of doing it in Next.js and, we can do it here by adding the Head in the _app.js file. Before proceeding further, you need to import the Fragment from the react library.
import '../styles/globals.css'
import Head from "next/head";
import {Fragment} from "react";
function MyApp({ Component, pageProps }) {
return (
<Fragment>
<Head>
<title>My Next App</title>
<meta description="Helle there. This is the Next.js App" />
</Head>
<Component {...pageProps} />
</Fragment>
)
}
export default MyApp
That's all. We want to test it before proceeding. Let's create a new route (/home) by creating a new file home.js under the page directory.
Add the following content:
function HomePage() {
return (
<div>
This is the Home Page
</div>
)
}
export default HomePage
It's time to visit the URL http://localhost:3000 and view the source page or examine the inspect element. You will see the title and description of the page.
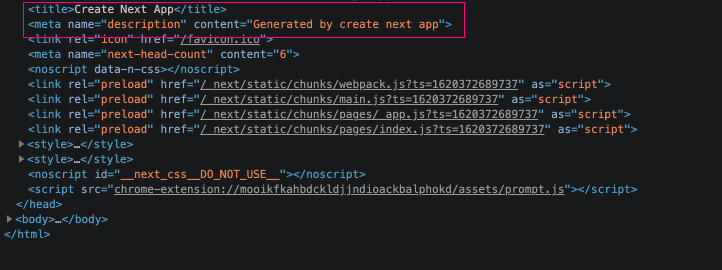
Here is another catch. If you visit the URL http://localhost:3000/ and do the inspect element. You can't find the title and description you added in the _app.js. Why? In Next.js, the Head tag in the route supersedes the Head tag from the _app.js file. It simply merges and updates the Head with the latest value. In this case, we defined the title in the index.js file. It updates the Head tag (from _app.js) with the title in the index.js file.
Lang Attribute and Meta description
At this point, if you generate a lighthouse report, you will see an error element does not have an [lang] attribute.
The lang attribute is essential for Search Engines. So it's always advisable to add the lang attribute.
To add the lang attribute, create a new file _document.js under the pages folder. The _docuement.js file manages the complete HTML document.
Unlike the _app.js file, it's a Class-based component.
import Document from "next/document";
import {Html, Head, Main, NextScript } from "next/document";
class AppDocument extends Document {
render() {
return(
<Html lang="en">
<Head>
<meta name="description" content="This is my App description."/>
</Head>
<body>
<Main></Main>
<NextScript></NextScript>
</body>
</Html>
)
}
}
export default AppDocument
If you regenerate the lighthouse report, you can see the SEO Score as 100%.
That's all for now. See you in the next article.
AK Newsletter
Join the newsletter to receive the latest updates in your inbox.