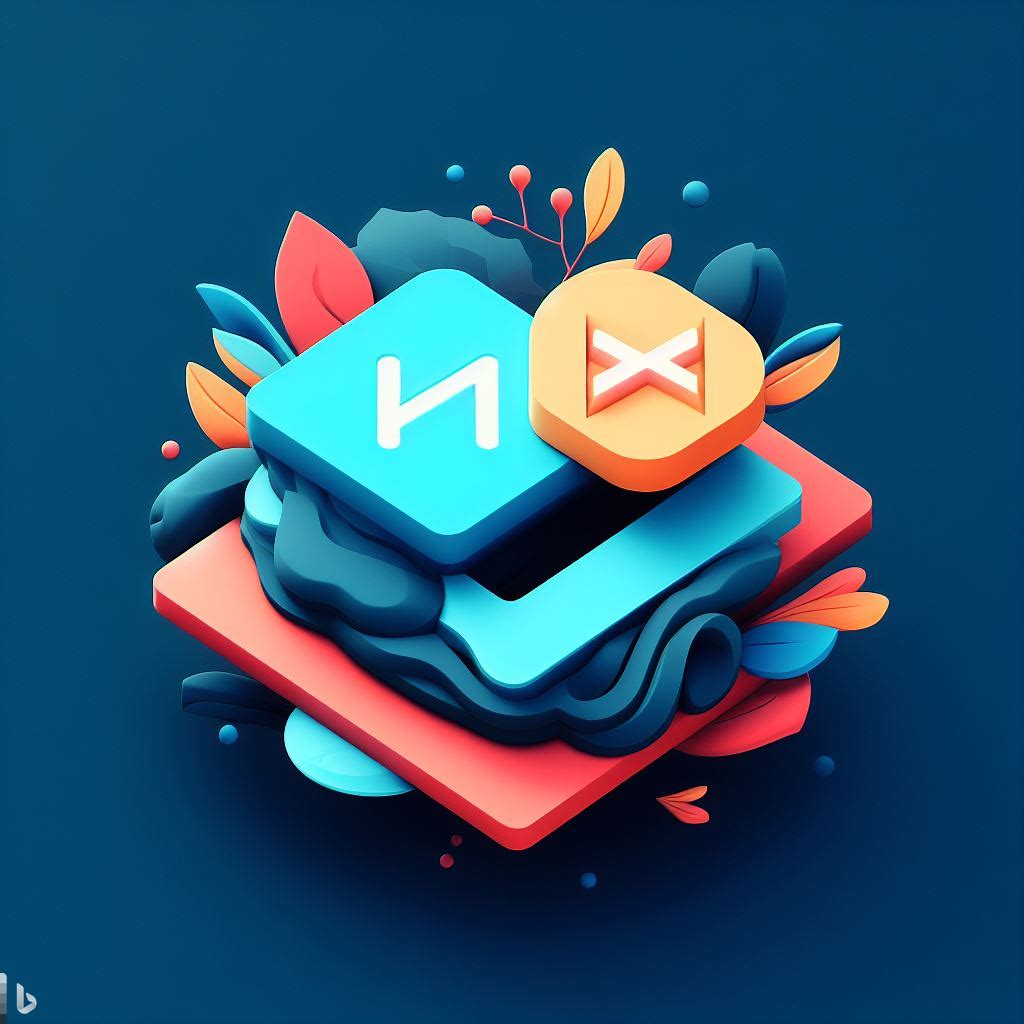
Building Dynamic Web Applications with Laravel and HTMX
In recent years, the way we build web applications has undergone a significant transformation. Traditional full-page reloads are giving way to dynamic, single-page applications that provide a smoother and more interactive user experience. Laravel, a popular PHP framework, has kept pace with these changes and offers seamless integration with modern front-end technologies like HTMX to build dynamic web apps.
What is HTMX?
HTMX is a powerful library that allows you to add dynamic behavior to your web applications without writing a lot of JavaScript. It's often described as "HTML over the wire" because it allows you to send HTML responses from the server to update parts of a page without reloading the entire thing. This is especially valuable in Laravel applications, as it aligns perfectly with Laravel's commitment to developer productivity.
In this tutorial, we'll explore how to use Laravel and HTMX to build a dynamic web application step by step.
Prerequisites
Before we start, make sure you have the following installed:
Setting Up a Laravel Project
If you haven't already, create a new Laravel project using Composer:
composer create-project laravel/laravel laravel-htmx-app
Installing HTMX
To use HTMX in your Laravel project, you can include it via a CDN in your Blade template or install it using npm. Let's go with npm:
npm install htmx
Creating a Simple Task List
Let's create a simple task list application. We'll start by creating a Task
model and a corresponding migration:
php artisan make:model Task -m
Next, open the migration file (database/migrations/yyyy_mm_dd_create_tasks_table.php
) and define the tasks
table schema:
public function up()
{
Schema::create('tasks', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->timestamps();
});
}
Migrate the database to create the tasks
table:
php artisan migrate
Now, let's create a controller and routes for managing tasks:
php artisan make:controller TaskController
Define routes in routes/web.php
:
Route::resource('tasks', TaskController::class);
Creating the Task List View
Create a Blade view for displaying the task list in resources/views/tasks/index.blade.php
:
@extends('layouts.app')
@section('content')
<h1>Task List</h1>
<ul id="task-list">
@foreach ($tasks as $task)
<li>
<span>{{ $task->name }}</span>
<button class="delete" data-hx-delete="{{ route('tasks.destroy', $task) }}" data-hx-confirm="Are you sure?">Delete</button>
</li>
@endforeach
</ul>
<form action="{{ route('tasks.store') }}" method="POST" id="task-form">
@csrf
<input type="text" name="name" placeholder="New Task" required>
<button type="submit">Add Task</button>
</form>
@endsection
n this view:
- We're using HTMX data attributes (
data-hx-...
) to define the dynamic behavior. - Each task item has a "Delete" button that sends a DELETE request to the server when clicked. HTMX handles this for us.
- We also have a form for adding new tasks.
Creating HTMX Actions
In the TaskController
, we'll define the actions for our dynamic task list:
use App\Models\Task;
use Illuminate\Http\Request;
public function index()
{
$tasks = Task::latest()->get();
return view('tasks.index', compact('tasks'));
}
public function store(Request $request)
{
Task::create(['name' => $request->input('name')]);
return back();
}
public function destroy(Task $task)
{
$task->delete();
return response()->noContent();
}
Adding HTMX Scripts
In your Blade layout file (resources/views/layouts/app.blade.php
), include the HTMX scripts at the end of the <body>
tag:
<script src="{{ asset('node_modules/htmx.org/dist/htmx.js') }}"></script>
Testing the Application
Start the Laravel development server:
php artisan serve
Visit http://localhost:8000/tasks
in your web browser. You can add and delete tasks without page
Visit http://localhost:8000/tasks
in your web browser. You can add and delete tasks without page reloads, thanks to HTMX.
Conclusion
In this tutorial, we've seen how to integrate HTMX with Laravel to create dynamic web applications. HTMX's "HTML over the wire" approach simplifies front-end development, allowing you to build interactive web apps with less JavaScript code. This combination of Laravel and HTMX provides a powerful and elegant way to enhance user experiences while maintaining developer productivity.
Give it a try in your Laravel projects, and you'll find that building dynamic, data-driven web applications has never been easier!
See you in the next article!
AK Newsletter
Join the newsletter to receive the latest updates in your inbox.